Modularity: A Pillar of Reliable Software Design
In software design, there are several principles aimed at creating reliable, robust, and maintainable software. One of the foundational principles is Modularity
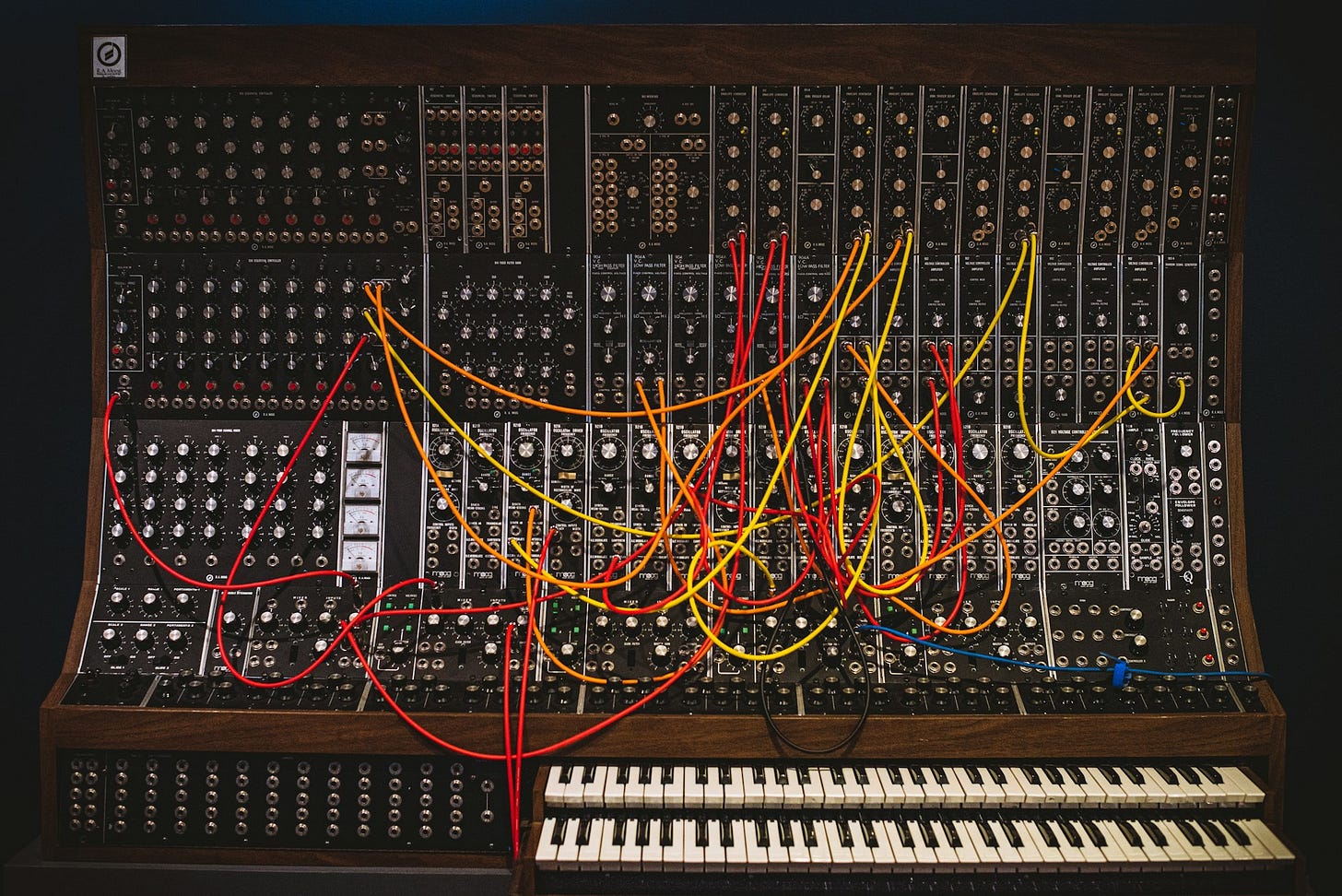
In our previous post we discussed principles of reliable software design, and in this post we will discuss the first pillar: Modularity
In software design, there are several principles aimed at creating reliable, robust, and maintainable software. One of the foundational principles is Modularity. This blog post will focus on understanding modularity, its benefits, and how it contributes to building reliable software systems.
Modularity is the principle of breaking down a complex system into smaller, manageable parts, or modules. Each module encapsulates a specific functionality and operates independently from the others. A complex problem can, therefore, be divided into smaller, more easily solved problems, each of which can be handled by a single module.
Benefits of Modularity
The key benefits of modularity are readability, maintainability, and reusability. A modular system is easier to understand because the functionality is split among distinct modules. It's also easier to maintain because changes to one module generally won't affect the others. Finally, well-designed modules are reusable, meaning the same code can be used in different parts of the application or even different applications.
Modularity in Practice
Consider a simple example of a web service using the Flask web framework in Python. In this service, we have two modules: a user module and a product module. The user module handles the registration and login of users, while the product module handles the listing and creation of products. By separating user-related functionality from product-related functionality, the code becomes easier to understand, maintain, and test.
Let's start with the user module. This module will handle the registration and login of users:
# file: user_module.py
from flask import Flask, request
user_app = Flask(__name__)
users = {}
@user_app.route('/register', methods=['POST'])
def register():
user_data = request.get_json()
username = user_data['username']
password = user_data['password']
users[username] = password
return {'message': 'User registered successfully'}, 200
@user_app.route('/login', methods=['POST'])
def login():
user_data = request.get_json()
username = user_data['username']
password = user_data['password']
if users.get(username) == password:
return {'message': 'Login successful'}, 200
else:
return {'message': 'Invalid username or password'}, 401
Next, let's look at the product module. This module will handle the listing and creation of products:
# file: product_module.py
from flask import Flask, request
product_app = Flask(__name__)
products = []
@product_app.route('/products', methods=['GET'])
def list_products():
return {'products': products}, 200
@product_app.route('/products', methods=['POST'])
def create_product():
product_data = request.get_json()
products.append(product_data)
return {'message': 'Product created successfully'}, 200
In a larger application, these modules might be in separate files in separate directories. They could also potentially be run as separate microservices or even Lambdas. But the main point is that the user-related functionality is separated from the product-related functionality, which makes the code easier to understand, maintain, and test.
In this case, the "Web Service" is divided into a "User Module" and a "Product Module". Each module can be developed and operated independently, while collectively they serve the entire web service.
Modularity and Software Reliability
Modularity significantly contributes to the reliability of software. When modules are well-designed and operate independently, a bug or failure in one module doesn't necessarily affect the others. This limits the impact of errors and makes it easier to identify and resolve them, leading to more robust and reliable software. Furthermore, each module can be independently tested and verified to ensure it correctly performs its intended function. This results in a higher overall system reliability.
Below are the ways in which modularity improve reliability:
Isolation of Faults: In a modular system, if a module fails, it does not necessarily cause the entire system to fail. The failure is often confined to that particular module. This isolation of faults means the rest of the system can continue operating, enhancing overall system reliability.
Simplified Testing and Debugging: Each module can be tested and debugged independently, which can lead to more thorough and efficient error checking. It's easier to locate and fix faults in a smaller, isolated piece of the system than in a large, monolithic system.
Easier Maintenance and Upgrades: Modularity allows individual components to be upgraded, repaired, or replaced without needing to disturb the entire system. This ease of maintenance can further improve reliability as problematic components can be addressed without impacting overall system performance.
Redundancy: In some modular systems, redundancy can be built in, meaning multiple modules can perform the same function. If one module fails, another can take over, thereby improving reliability.
Better Quality Control: With modularity, each component can be built and tested to its own strict standards, often leading to higher overall quality of the system.
Parallel Development: Modular design allows different parts of a system to be developed concurrently. This parallel development can lead to more thorough design and testing, which can in turn improve the reliability of the system.
In the next post, we'll explore the principle of Loose Coupling and how it interacts with Modularity to create more robust and scalable systems. Stay tuned!